Introduction
A solid understanding of data structures and algorithms is crucial for programmers to enhance problem-solving skills in computer science. On other side, interviewers often use DSA questions to evaluate coding skills, problem-solving skill, and clarity of thought.
So one idea is clear: Knowledge of algorithms and data structures is not only important for growing as a smart programmer but also for achieving dream success in tech industry. If you want to master DSA, following a well-defined guided learning plan is important, especially if you are a beginner with basic programming skills.
At the start of the learning journey, you may have questions like this:
- How can I develop a long-term interest in DSA?
- How do I create a continuous learning plan?
- Which topics are good to start the learning journey?
- What are the most crucial DSA concepts and problem-solving approaches?
- What are some key activities that will help me learn effectively?
- How can I prepare for a coding interview in a short time?
If you are looking for answers to these questions, then you have come to the right place. Let's start step by step.
Step 1: Developing long-term motivation
Learning and mastering data structures and algorithms require motivation and perseverance. Here are some thoughts that can keep you motivated on your journey:
- The ability to design efficient algorithms is a highly valued skill in programming, and mastering it can lead to great career opportunities.
- Never get discouraged by setbacks. Once you grasp the basics, your DSA problem-solving skills will improve tremendously.
- Never give up! If you encounter a difficult problem, challenge yourself to give it one more try. These moments can be defining points in your DSA journey.
- Mastering coding and problem-solving is a continuous process that involves learning from mistakes. Each error or bug presents an opportunity to gain new insights and improve your skills.
Blog to explore: Why should we learn Data Structures and Algorithms?
Step 2: Understanding data structures and algorithms syllabus
Now, our goal should be to prepare a list of important topics. To help you with this, we are sharing here a comprehensive list of DSA topics to learn in a defined order. Note: Never forget to explore real-world applications to understand how algorithms are used in practice.
1. Algorithmic and mathematical thinking
Important topics: Algorithmic puzzles, Brainteaser puzzles, Counting, Summation, Numbers theory, Permutation, Mathematical induction, Recurrence relations, Bit manipulation, Probability, etc.
Blog to explore: How to develop algorithmic thinking?
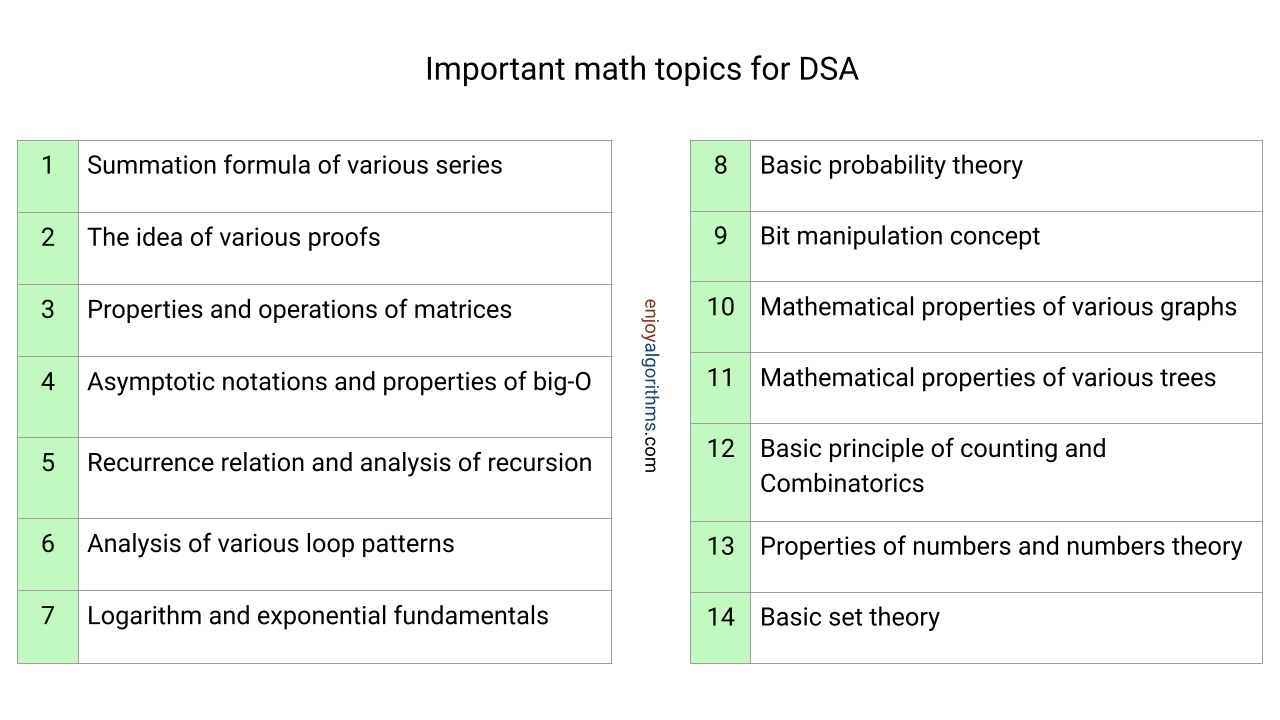
2. Fundamentals of programming
Important topics: Data types, Variables, Operators, Expressions, Control statements, Loop, Function, Pointers and References, Array, String, Memory management, Fundamental of OOPS, etc.
3. Fundamental of algorithm
- Algorithm introduction: Properties and Real-Life Applications of Algorithms.
- Complexity analysis: Input size, Rate of growth, Time complexity, Big-O notations, Worst-case analysis, Best-case analysis, Average-case analysis, Space complexity analysis, etc.
- Iteration: Properties of iteration, Analysis of loop, Patterns of iterative problem-solving such as building partial solutions using single loop, Nested loop, Two pointers, Sliding window, etc.
- Recursion: Properties of recursion, Analysis of recursion, Patterns of recursive problem-solving such as decrease and conquer, Divide and conquer, etc.
- Sorting: Bubble sort, Selection sort, Insertion sort, Merge sort, Quick sort, Heap sort, Counting sort, Radix sort, Properties and comparisons of sorting algorithms, etc.
- Searching: Binary search, Exponential search, Interpolation search, etc.
- Introduction to data structures: Properties, Types, Key operations, Concepts of abstract data types, Real-life applications.
4. Linear data structures
- Array: 1D array, 2D array, string, dynamic array
- Linked list: Singly linked list, doubly linked list, circular linked list.
- Stack, queue, and dequeue.
5. Non-linear data structures
- Hashing: Direct address table, Hash table, Properties and design of a good hash function, Basic operations (insert, delete, and search), Patterns of problem-solving using hash table, Collision resolution techniques like chaining and open addressing, etc.
- Binary tree: Properties and structure, Recursive DFS traversals, Iterative DFS traversal, BFS traversal, Basic operations, Patterns of problem-solving using BFS and DFS traversals, etc.
- Binary search tree: Properties and structure, BST building, BST operations, BST sort, Patterns of problem-solving in BST, Self-balancing BST, Real-life applications, Comparison with hash table.
- Heap: Properties and structure, Heap building, Basic heap operations, Heap sort, Patterns of problem solving, Real-life applications.
- Dictionary and Priority queue: Implementation using array, linked list, BST, Heap (priority queue) and Hash Table (Dictionary), Time complexity comparisons of basic operations, and Real-life applications.
- Advanced data structures (Properties, structure, basic operations, patterns of problem-solving and Real-life applications): Trie, Segment tree, Binary indexed tree, K-dimensional tree, n-ary tree, Suffix tree.
- Graph: Properties, Structure, Types, Adjacency matrix representation, Adjacency list representation, BFS traversal and its properties, DFS traversal and its properties, Problem-solving patterns using DFS and BFS, Topological sorting, Shortest path algorithms, Minimum spanning tree, etc.
6. Algorithm design techniques
- Dynamic programming: Time memory tradeoff, optimization and combinatorial problems, Designing recursive solution of a DP problem, Top-down approach, Bottom-up approach, Problem-solving patterns, Divide and conquer vs Dynamic programming, etc.
- Greedy algorithms: Greedy choice property, Problem solving patterns, DP vs Greedy algorithms, etc.
- Backtracking: Exhaustive search, Recursive and iterative backtracking, Branch and bound, Bit-masking, etc.
- String algorithms: String matching, Patterns of problem solving, etc.
- Mathematical algorithms: Numbers theoretic algorithms, Bitwise algorithms, Randomised algorithms, etc.
7. Coding interview preparation
- Continuous practice of coding questions
- Profile and resume building
- Preparation via mock Interview
- Company-specific research of interview process
- Behavioral interview preparation
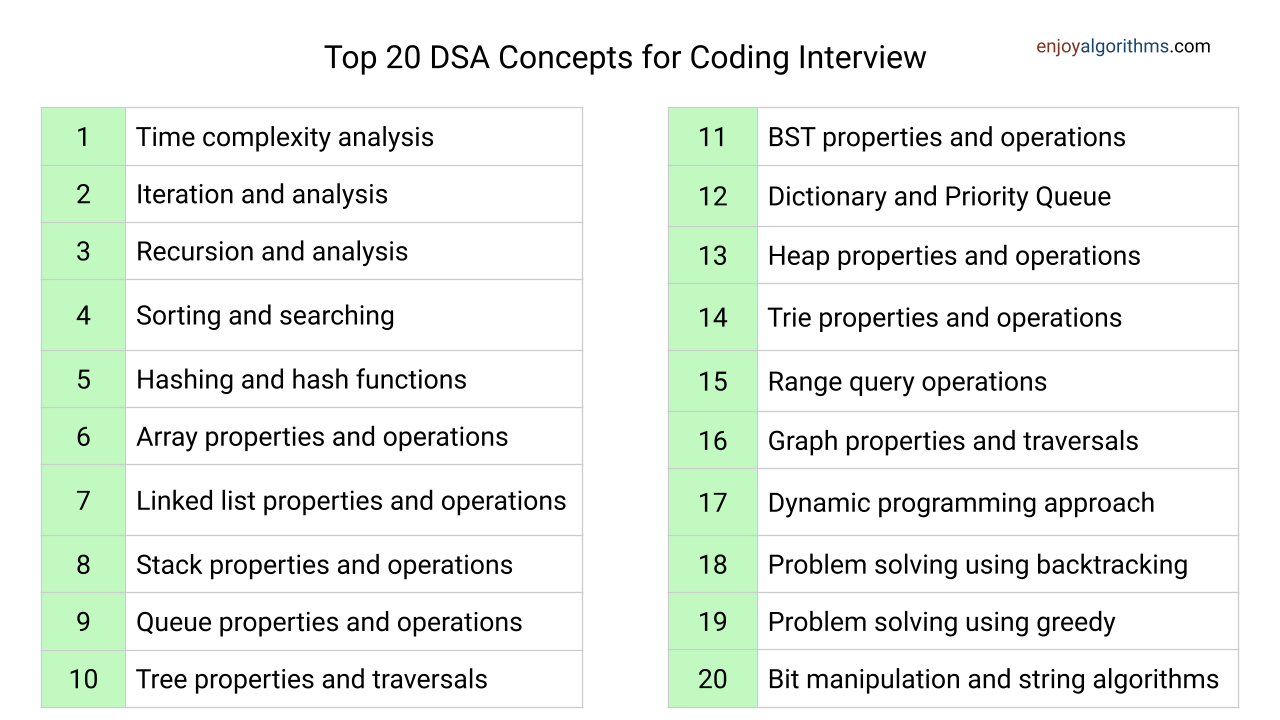
Step 3: Collecting the best resources for learning and practice
Our next step should be to gather one of the best learning resources: books, blogs, talks, PDFs, courses, etc. The internet can be a great tool for this. Note: It's important to follow authentic sources and organize them according to the syllabus we've outlined. Here is the list of some best learning resources:
Data structures and algorithms resources for beginners
- Book: Algorithms Unlocked by Thomas Coreman, MIT Press
- Book: Algorithmic Puzzles by Anany and Maria Levitin, Oxford University Press
- Course: Mathematics for computer science by MIT Open Courseware
Resources for learning basic and advanced programming concepts
- Book: Think Python (How to Think Like a Computer Scientist)
- Book: C++ Primer
- Book: Introduction to Programming in Java
- Course: Programming Paradigms by Stanford
- Course: Programming Abstraction by Stanford
- Course: Programming Methodology by Stanford
- Book: Clean Code by Robert Martin
Data structures and algorithms resources for advanced learners
- Algorithms by CLRS (Book): Complexity analysis, Divide and conquer, Sorting, Linear data structures, Hash table, Heap, BST, Graph, Dynamic programming, Greedy algorithms, String algorithms.
- The Algorithm Design Manual by Steven Skiena (Book): Complexity analysis, Sorting and Searching, Linear and Non-linear data structures, Backtracking and Dynamic programming.
- Algorithms by Robert Sedgewick (Book): Complexity analysis, Sorting, Stack, Queue, Priority queue, Hash table, Binary search tree, Graph, String algorithms.
- Course: Introduction to Algorithms by MIT Open Courseware.
For competitive programming
For data structures and algorithms interview
Step 4: Finding good mentors and communities
If you want to take your DSA skills to the next level, having a good mentor can be invaluable. An experienced mentor can guide you in building a strong foundation in data structures, mastering problem-solving strategies, clarifying doubts, and preparing for coding interviews.
Additionally, forming a study group with like-minded individuals can be a great idea. This provides an excellent opportunity to learn from each other, support one another, and work towards a common DSA goals. You can also join online communities or groups to learn from experiences of others and seek help with coding questions.
Step 5: Preparing a continuous learning plan for coding Interview
This is one of the critical steps in learning data structures and algorithms. We recommend dedicating minimum of 10 hours per week and designing your plan based on your personal learning goals. Finding a balance that works for you is key, so make sure to stick to your plan consistently.
4-Week quick revision and coding interview preparation plan
This is recommended for learners who want to refresh their concepts and problem-solving for an incoming coding interview. Such learners should have basic problem-solving skills in data structure and algorithms and good knowledge of programming concepts.
- Minimum estimated time = 15 x 4 = 60 hours (Such learners should try to give atleast 15 hours per week).
- Minimum coding problems to practise = 50 (These questions should cover all popular approaches in DSA).
- Concept revision and problem-solving research = 20 hours.
- Problem-solving on paper with pseudocode = 20 Hours (At least 40 coding questions).
- Problem-solving with coding practice = 10 hours (At least 10 coding questions).
- Behavioural interview preparation + Mock interviews = 10 Hours.
8-Week revision and coding interview preparation plan
This is recommended for learners who want to revise DSA concepts and sharpen problem-solving skills for an incoming coding interview. Such learners should have basic problem-solving skills in data structure and algorithms and good knowledge of programming concepts.
- Minimum estimated time = 10 x 8 = 80 hours.
- Minimum coding problems to practise = 75.
- Concept revision and problem-solving research = 20 hours.
- Problem-solving on paper with pseudocode = 25 Hours (at least 50 coding questions).
- Problem-solving with coding practice = 25 Hours (at least 25 coding questions).
- Behavioural interview preparation + Mock interviews = 10 Hours.
16-Week learning and coding interview preparation plan
This is recommended for intermediate learners who want to learn DSA concepts and sharpen problem-solving skills. Such learners should have a basic understanding of programming concepts.
- Minimum estimated time = 10 x 16 = 160 hours.
- Minimum coding problems to practise = 150. (This list of questions should cover problems with various difficulties level).
- Concept learning/revision and problem-solving research = 40 hours.
- Problem-solving on paper with pseudocode = 50 hours (at least 100 coding questions).
- Problem-solving with coding practice = 50 hours (at least 50 coding questions).
- Behavioural interview preparation + Mock interviews = 20 hours.
24-Week learning and coding interview preparation plan
This is recommended for beginners who want to learn DSA from scratch and prepare for interview.
- Minimum estimated time = 10 x 24 = 240 hours.
- Minimum coding problems to practise = 150.
- Learning basic programming and math for DSA = 20 hours.
- Concept learning and problem-solving research = 80 hours.
- Problem-solving on paper with pseudocode = 60 hours (at least 100 coding questions).
- Problem-solving with coding practice = 60 hours (at least 50 coding questions)
- Behavioural interview preparation + Mock interviews = 20 hours.
Step 6: Starting continuous learning activities
Concept learning and revision
To fully understand DSA concepts, it's crucial to learn them in the right order. You should create self-notes and use visuals to organize your ideas. This will help you in revising the concepts later. It's also a good idea to brainstorm with a friend to discuss each topic and to seek guidance from a mentor when needed.
Problem-solving research
Improving your DSA skills can be achieved by exploring patterns through various coding questions. It's important to identify important problem-solving strategies and their use cases, just like reading solutions and making notes about the thought process.
Before diving into problem-solving, we should explore various examples to understand the application of concepts. So we recommend taking the time to thoroughly analyze different approaches by going through the solution of at least 3-4 coding questions. This will help you gain a better understanding of different techniques.
Blog to explore: Popular Problem-Solving Approaches in Data Structures and Algorithms
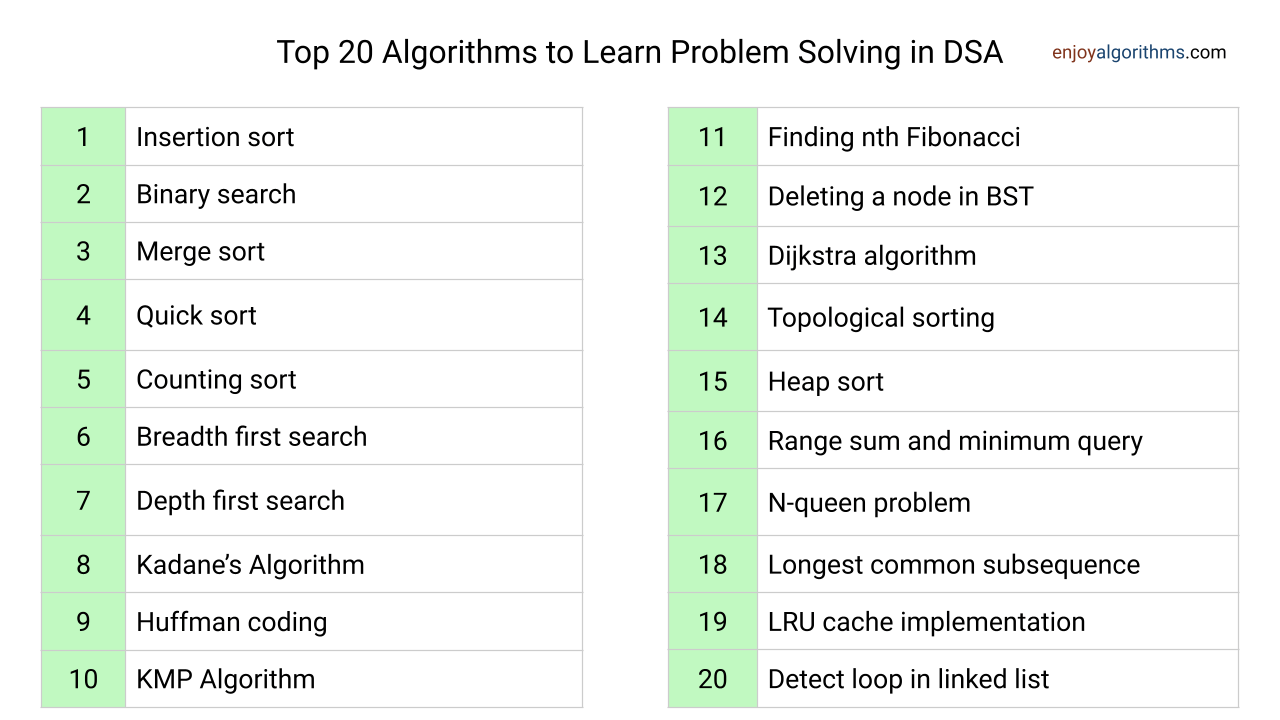
Problem-solving on paper and coding practice
Practicing DSA problem-solving skills on paper or a whiteboard can be really helpful. Visualizing solutions, creating pseudo-codes, and outlining the steps of your algorithms can help you design more efficient and effective solutions. Using paper or a whiteboard is great because it allows you to easily sketch out your ideas and make changes as needed.
One more thing: There are a lot of problems available on the internet, so we need to choose some of the popular interview questions that can be solved using various approaches.
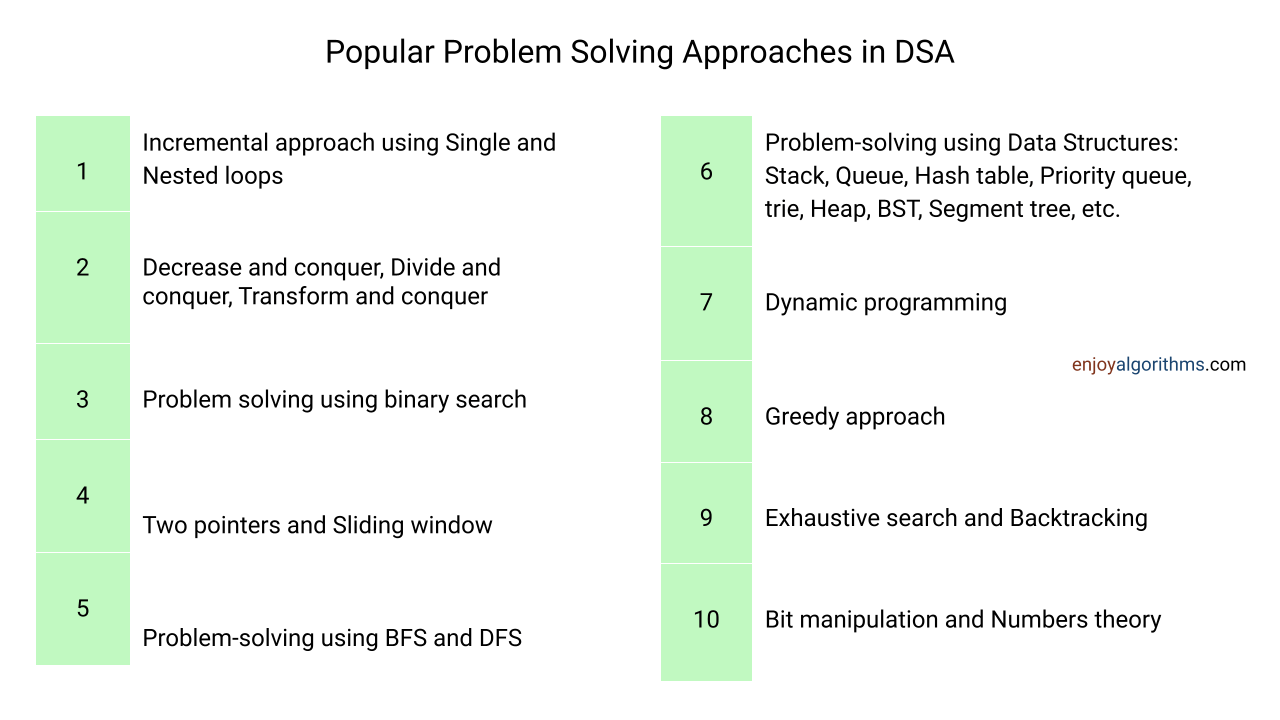
After designing pseudo-code, we should work to translate it into actual code using the programming language of your choice. It's also important to thoroughly test your code using a variety of input and output data, edge cases, and boundary conditions to make sure it's working correctly. We need to think:
- Does the code produce correct output for all possible inputs?
- Can we optimize the code further? It's always a good idea to improve efficiency of your code. If you have time, take another look at the solution and see if there's a better way to implement it.
- How can we improve the code readability? Making sure your code is well-organized and easy to understand is important for you and anyone else who might need to read and maintain it in the future.
Blog to explore: Steps of problem-solving in Data Structure and Algorithms
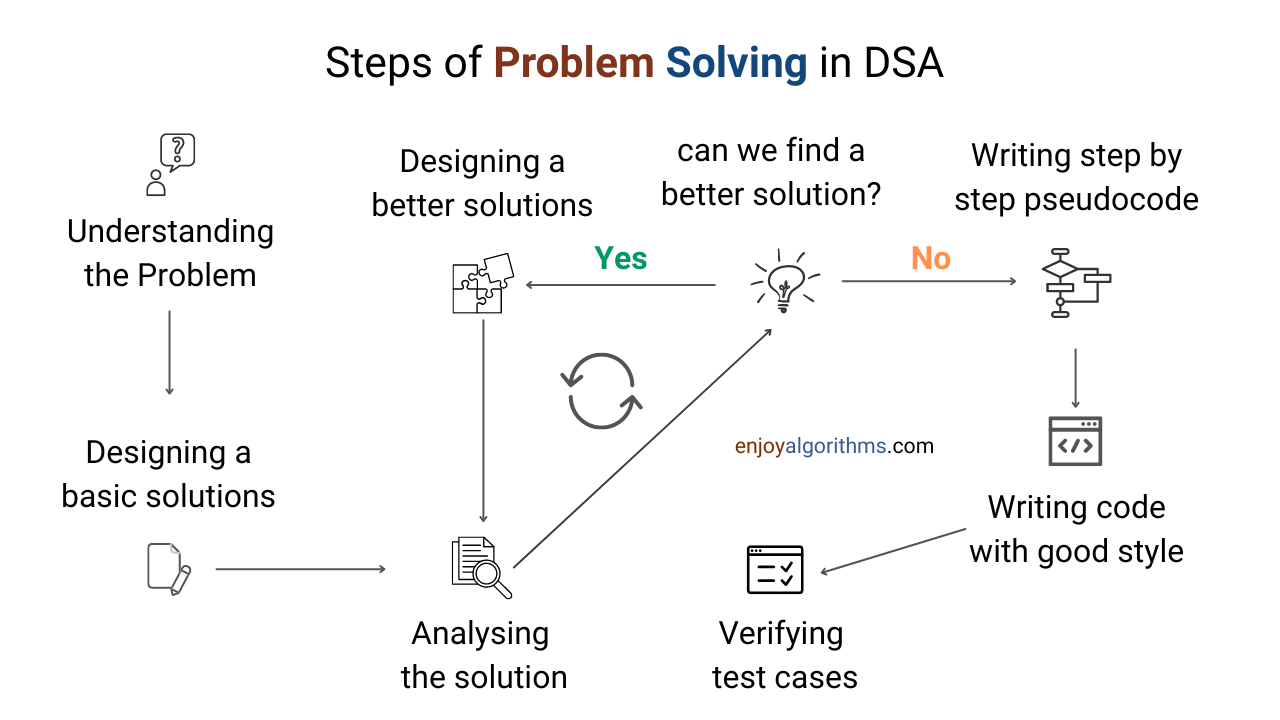
Resolving critical doubts
It's totally normal to have questions and doubts when you're learning algorithms and data structures. But don't let these doubts hold you back! Use them as opportunities to deepen your understanding and take your learning to the next level. If you're struggling to resolve a doubt, there are few ways to get some help:
- Talk to a friend or colleague about it.
- Ask a mentor or course instructor for guidance.
- Post your question in a coding or learning community.
- Make a note of the doubt and try it later with a fresh perspective. Sometimes, doubts may be related to concepts that you haven't learned yet, and it can be helpful to revisit them once you have more context.
Don't be afraid to ask for help. We all have to start somewhere, and seeking guidance is a great way to move forward and continue learning.
Interview preparation via mock interviews
Mock interviews are one of the best ways to get ready for the big day. Find a partner who shares similar career goals and conduct mock interviews with each other. This will help you get feedback on your performance and identify areas where you can improve.
- When selecting a partner, ensure they have a basic understanding of data structures and algorithms.
- During the mock interview, use a timer to keep the session to about 1 hour or 45 minutes, which is the average length of a real coding interview.
- To make the mock interview as realistic as possible, properly prepare your partner and follow all necessary steps. Don't forget to switch roles after the mock interview and take on the role of the interviewer. This will give you some insight into what companies are looking for in a candidate.
Mock interviews aren't just helpful for feedback, but they can also improve your body language and communication skills. Consider recording the session and reviewing the footage to identify areas for improvement.
Behavioral interview preparation
Behavioral interviews are a critical part of the job interview process, particularly for programmers aspiring to secure their dream job. Surprisingly, many programmers tend to overlook the importance of behavioral interviews and fail to prepare for them adequately. It's vital to understand that behavioral interviews hold just as much significance as technical interviews.
In a behavioral interview, the interviewer assesses how you approach decision-making and handle challenging situations. This evaluation is crucial as it helps the interviewer determine whether you're the right fit for the company and the position. Therefore, investing time and effort into preparing for behavioral interviews is essential. Demonstrate your skills and abilities, and you'll be one step closer to securing your dream job!
The idea of behavioral interviews can vary from company to company, depending on their values and culture. Here are some tips to keep in mind:
- Practice good communication skills
- Read the job description more than once (the more the better!)
- Research the industry and company
- Prepare for common questions
- Make your sales pitch clear
- Pay attention to your body language
- Come up with smart questions for the interviewers
- Be positive, authentic, and truthful in your answers
- Stay optimistic and embrace the challenge
It's also a good idea to have at least one strong example of:
- An interesting technical problem you solved
- An interpersonal conflict you overcame
- A unique execution story about a past project
- Leadership or ownership
- A question about the company's product/business
- A question about the company's engineering strategy
Tips for Learning Data Structure and Algorithms
Here are some tips to help you get the most out of your data structures and algorithms journey:
- Aim to cover at least 80% of the syllabus and prioritize topics based on your strengths and weaknesses. If you find a topic challenging, don't be afraid to spend more time on it.
- Conduct a weekly critical review to keep track of your progress. Evaluate each topic and ask yourself what you need to do next to improve. You can rate your understanding of each topic on a scale of 1 to 10 for a more objective view.
- Take note of any unique or essential patterns you encounter as you learn DSA, such as brute force and efficient solutions, boundary conditions, time and space complexity analysis, and coding style.
- Instead of trying to solve a lot of problems, focus on the top 150 coding interview questions in the beginning. Choose some well-known coding problems and try to solve them using different techniques.
- Visualize critical operations, implementations, and use cases for each data structure to improve your understanding.
- Don't hesitate to revisit the same concepts and problems multiple times. This can help you uncover hidden ideas. Focus on understanding the reasoning behind things rather than trying to memorize everything.
Tips for cracking the coding interview
To ace your coding interviews, having a well-defined strategy is crucial. It not only boosts your confidence but also gives you an edge over the competition. Here are some key tips to remember:
- Write down your thoughts and visualize the problem on paper. Create test cases and write pseudocode to map out your solution.
- Don't focus on designing the optimal solution right away. Start with a brute force approach and refine it.
- Communication is key. Keep the interviewer in the loop on your approach and any challenges you face.
- Remember that you're working as part of a team to solve the problem. Collaboration skills are just as important as technical skills.
- Don't get discouraged if you get stuck. The interviewer wants to see how you approach problem-solving.
- Think out loud and explain your thought process. The interviewer may provide hints based on your approach.
- Look for popular problem-solving patterns and apply them as needed.
- Use descriptive variable names and follow good coding practices.
- Test your solution thoroughly, including edge cases.
A final motivation!
Mastering data structures and algorithms require a long-term vision, hard work, and consistency. Keep in mind this inspiring quote: "If the mind is weak, then the situation becomes a problem; if the mind is balanced, then the situation becomes a challenge. But if the mind is strong, then the situation becomes an opportunity."
Learning and coding can be enjoyable, so it's essential to embrace the process and have fun! With the right mindset and dedication, you can succeed in your DSA learning journey and make the most of the opportunities that come your way. Best of luck!
If you have any queries/doubts/feedback, please write us at contact@enjoyalgorithms.com. Enjoy learning, enjoy coding, enjoy algorithms!